Are you looking to test your programming knowledge with Programming MCQs? If so, you’ve come to the right place! We’ve compiled a list of Multiple Choice Questions for Programmers to help you better understand the nuances of programming and stay on top of your game. These questions cover a wide range of topics, from basic coding concepts to more advanced programming techniques. Whether you’re an experienced programmer or just starting out, these MCQs can help you gain a better understanding of the programming world. So, let’s get started and see how much you know about programming!
Here are some Programming MCQ covering various topics:
Which of the following is not a high-level programming language? a) C++ b) Python c) Assembly Language d) Java Answer: c) Assembly Language
2. What is the output of the following code snippet? a = 5 b = 3 print(a % b)
a) 1 b) 2 c) 3 d) 4 Answer: a) 2
3. Which of the following is not a basic data type in Python?
a) int
b) float
c) char
d) bool
Answer: c) char
4. What is the output of the following code snippet?
for i in range(3):
for j in range(2):
print(i, j)
a) (0, 0) (0, 1) (1, 0) (1, 1) (2, 0) (2, 1) b) (0, 0) (1, 0) (2, 0) (0, 1) (1, 1) (2, 1) c) (0, 1) (0, 2) (1, 1) (1, 2) (2, 1) (2, 2) d) (1, 1) (1, 2) (2, 1) (2, 2) (3, 1) (3, 2) Answer: b) (0, 0) (1, 0) (2, 0) (0, 1) (1, 1) (2, 1)
5. Which of the following sorting algorithms has the worst-case time complexity of O(n^2)? a) QuickSort b) MergeSort c) InsertionSort d) HeapSort Answer: c) InsertionSort
6. What is the output of the following code snippet?
def foo(x):
if x == 0:
return 0
else:
return x + foo(x-1)
print(foo(5))
a) 10 b) 15 c) 20 d) 25 Answer: b) 15
7. Which of the following data structures is not a linear data structure? a) Array b) Linked List c) Tree d) Stack Answer: c) Tree
8. What is the output of the following code snippet?
x = [1, 2, 3, 4, 5]
y = x[1:4]
print(y)
a) [1, 2, 3] b) [2, 3, 4] c) [2, 3, 4, 5] d) [1, 2, 3, 4] Answer: b) [2, 3, 4]
9. Which of the following operators in Python is used to concatenate two strings?
a) +
b) *
c) /
d) –
Answer: a) +
10. What is the output of the following code snippet?
a = [1, 2, 3, 4, 5]
b = list(filter(lambda x: x%2 == 0, a))
print(b)
a) [2, 4] b) [1, 3, 5] c) [1, 2, 3, 4
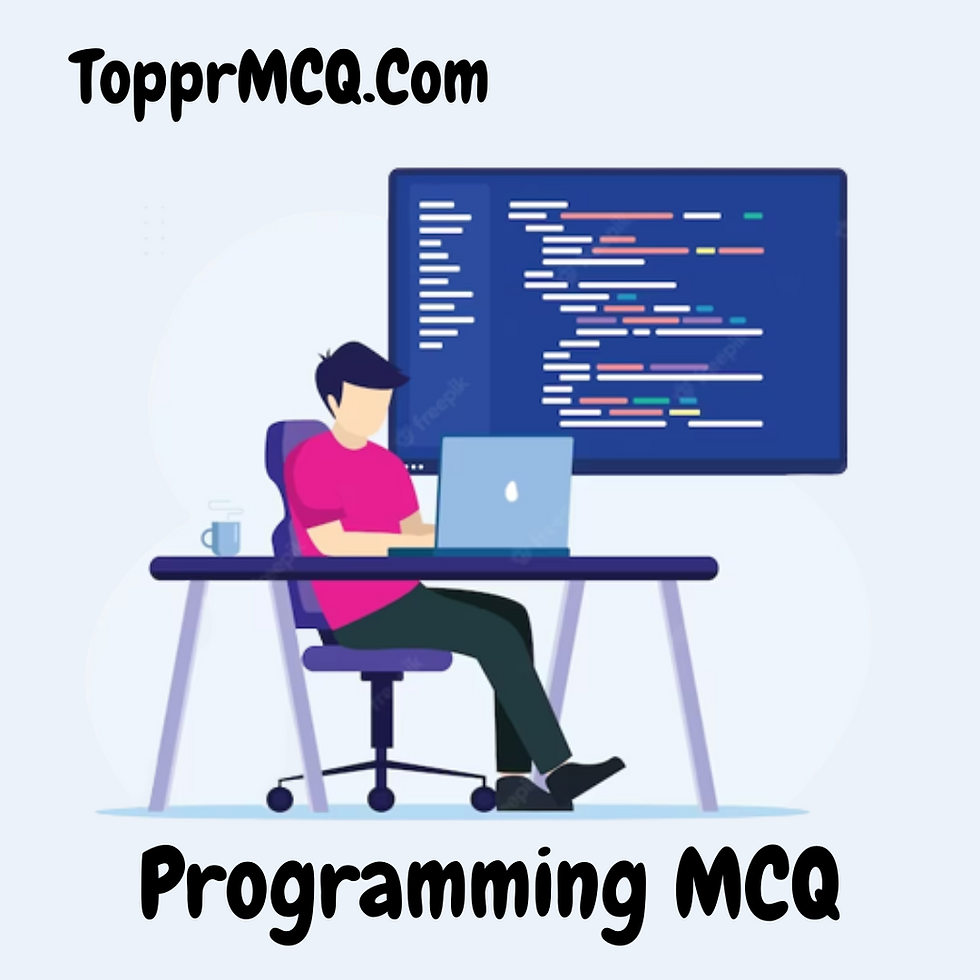
Here’s an outline suggestion for Programming MCQs:
I. Introduction
Definition of programming and its importance
Brief explanation of MCQs and their use in testing programming concepts
II. Basic Concepts of Programming
Variables and Data Types
Operators
Control Structures
Functions and Procedures
III. Object-Oriented Programming
Classes and Objects
Inheritance and Polymorphism
Abstraction and Encapsulation
IV. Data Structures
Arrays
Linked Lists
Stacks and Queues
Trees and Graphs
V. Algorithms
Sorting Algorithms (Bubble Sort, Insertion Sort, Selection Sort, Merge Sort, Quick Sort)
Searching Algorithms (Linear Search, Binary Search)
Recursion
Dynamic Programming
VI. Advanced Topics in Programming
Exception Handling
File Handling
Regular Expressions
Multithreading
Networking
VII. Conclusion
Summary of programming concepts covered in the MCQs
Importance of continuing to learn and practice programming skills
Note: The number and scope of MCQs can be adjusted based on the level and purpose of the test.
Here are some SEO keyword-rich titles related to Programming MCQ:
Test Your Programming Knowledge: Programming MCQs for Beginners
Advanced Programming Concepts: Multiple Choice Questions (MCQs)
Object-Oriented Programming MCQ: Test Your Understanding
MCQs for Data Structures: Practice Your Programming Skills
Algorithm MCQs: Multiple Choice Questions for Sorting and Searching
Advanced Topics in Programming MCQ: Test Your Knowledge
Java Programming MCQs: Multiple Choice Questions for Java Developers
Python Programming MCQ: Practice Test Questions for Programmers
Web Development MCQ: Multiple Choice Questions for Front-End and Back-End Developers
Machine Learning MCQ: Test Your Understanding of Machine Learning Concepts
Note: These titles can be adjusted and customized based on the level and purpose of the Programming MCQ test.
I. Introduction
Programming is an essential skill in today’s digital age, and it’s becoming increasingly important to learn and practice programming concepts to stay competitive in the job market. Multiple choice questions (MCQs) are a common way to test programming knowledge and assess programming skills. Programming MCQs can cover a wide range of topics, from basic programming concepts like variables and data types to advanced topics like object-oriented programming, data structures, and algorithms. By testing your programming knowledge with MCQs, you can identify your strengths and weaknesses, gain more confidence in your programming abilities, and improve your overall understanding of programming concepts. In this context, we will explore some categories of programming MCQs and suggest some SEO keyword-rich titles to help you improve your programming skills and knowledge.
II. Basic Concepts of Programming
Basic concepts of programming include the fundamental building blocks that are used to create software. These concepts include variables and data types, operators, control structures, and functions/procedures. Variables are used to store and manipulate data in a program, and data types specify the kind of data that can be stored in a variable. Operators are used to perform various operations on data, such as arithmetic operations or comparisons. Control structures allow for the flow of a program to be controlled based on certain conditions or loops, enabling programs to make decisions and perform actions based on the data and other factors. Functions and procedures are blocks of code that can be reused throughout a program, increasing efficiency and readability. Understanding the basic concepts of programming is crucial to building more complex programs and understanding how programming languages work.
III. Object-Oriented Programming
Object-oriented programming (OOP) is a programming paradigm that is based on the concept of objects. In OOP, an object is an instance of a class, which is a template or blueprint that defines the properties and behaviors of an object. Classes can be used to create new objects with similar properties and behaviors, making OOP a powerful way to model complex systems. OOP provides four fundamental concepts: encapsulation, inheritance, polymorphism, and abstraction. Encapsulation refers to the practice of keeping the internal details of an object hidden, making it easier to maintain and change the code. Inheritance allows a class to inherit properties and behaviors from a parent class, while polymorphism enables objects to take on multiple forms or behaviors. Abstraction involves the use of abstract classes and interfaces to define common behaviors and properties that can be inherited by multiple classes. OOP is widely used in programming languages such as Java, C++, and Python, and is a crucial concept for building complex applications and systems.
IV. Data Structures
Data structures are a way of organizing and storing data in a computer program so that it can be accessed and used efficiently. Common data structures include arrays, lists, stacks, queues, trees, and graphs. Arrays are a collection of elements of the same data type, while lists allow for the insertion and deletion of elements at any point in the list. Stacks and queues are used to manage collections of data in a particular order, with stacks following the last-in, first-out (LIFO) principle and queues following the first-in, first-out (FIFO) principle. Trees and graphs are used to represent hierarchical and non-linear relationships between data elements. Data structures are used to solve a wide range of problems in computer science and are an essential concept for any programmer to understand. By choosing the appropriate data structure, programs can perform operations more efficiently, use less memory, and be easier to maintain and modify.
V. Algorithms
An algorithm is a set of instructions for solving a particular problem or completing a particular task. Algorithms can be expressed in many different ways, including natural language, pseudocode, and programming languages. They can be used to solve a wide range of problems, from simple mathematical calculations to complex machine learning models. Algorithms can be classified based on their time and space complexity, with the most efficient algorithms using the least amount of time and space. Common algorithmic techniques include searching, sorting, and graph traversal. Searching algorithms are used to find a particular value in a set of data, while sorting algorithms are used to put data into a particular order. Graph traversal algorithms are used to explore graphs or networks, identifying paths between nodes and determining the shortest path between two points. Understanding algorithms is an essential concept for any programmer, as it allows them to write efficient, optimized code that can handle complex tasks and large amounts of data.
VI. Advanced Topics in Programming
Advanced topics in programming refer to the more complex and specialized areas of programming that require a deeper understanding of programming concepts and techniques. These topics often require experience with programming languages, frameworks, and libraries.
Some examples of advanced topics in programming include:
Algorithm design and analysis: The study of algorithms and their efficiency in terms of time and space complexity.
Data structures: The organization and storage of data in memory or on disk, such as arrays, linked lists, trees, and graphs.
Parallel and concurrent programming: The design and implementation of programs that can execute multiple tasks simultaneously or in parallel.
Machine learning and artificial intelligence: The use of algorithms and statistical models to analyze data, learn from it, and make predictions or decisions.
Distributed systems: The design and implementation of software that runs on multiple computers and communicates over a network.
Security: The design and implementation of programs that are secure and protect against threats such as hacking and data breaches.
Compiler design: The design and implementation of software that translates programming languages into machine code.
To become proficient in advanced topics in programming, programmers often require years of experience, additional training, and a willingness to continue learning and staying up-to-date with the latest developments in the field.
VII. Conclusion
In conclusion, programming is a vast and dynamic field that requires continuous learning and adapting to new technologies and programming languages. Whether you are a beginner or an experienced programmer, it is important to stay up-to-date with the latest developments and trends in programming. The advanced topics in programming, such as algorithm design, data structures, machine learning, and security, require a deep understanding of programming concepts and techniques. To become proficient in these areas, it is essential to invest time and effort in learning and practicing. With dedication and hard work, anyone can become a skilled programmer and contribute to the development of innovative software solutions that can solve complex problems and improve our daily lives.
Comments